Accessing simple-auth protected websites from ESP8266
Not all pages are publicly available, sometimes, I have to access the password-protected page from my ESP. I will explain this in this article on the simple-auth-protected (Basic Authorization) page.
In such a case, you must send your username and password to the server. Simple auth requires this to be provided in the communication header. Here is how to prepare the data and send it:
First, you have to write down your username and password like this:
username:password
Please notice the colon “:” in between username and password. Next, you have to encode this to Base64, for instance, by using this site:
https://www.base64encode.org/ – you have to encode your whole string into Base64. Here is what it looks like with my example from above:
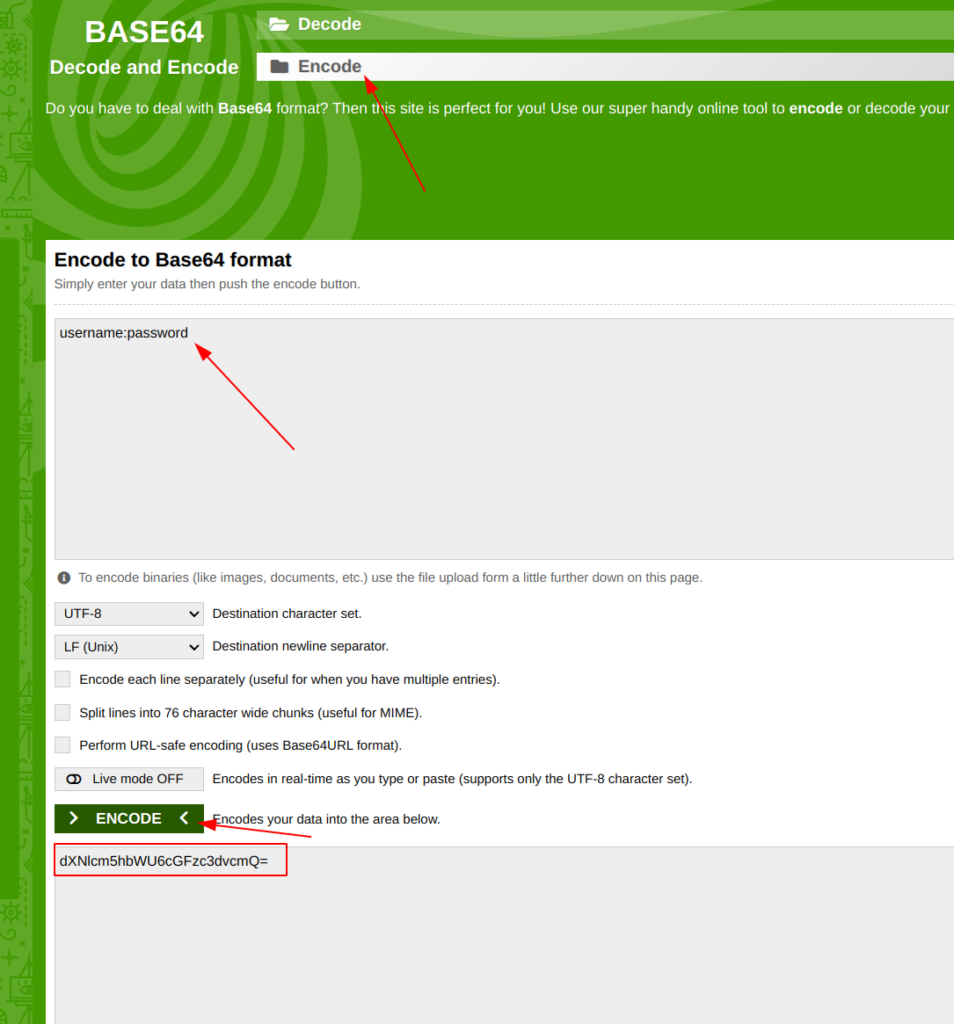
So, my Base64 encoded string is:
dXNlcm5hbWU6cGFzc3dvcmQ=
Lastly, In your HTTP request, you have to add the following header:
httpClientGetRequest.println("Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=");
Here is what it looks like in the context of communication:
httpClientGetRequest.println("GET " + URLpartToReadFromHost + " HTTP/1.1"); httpClientGetRequest.print("Host: my.website.host.name.com"); httpClientGetRequest.println("User-Agent: ESP8266/1.0"); httpClientGetRequest.println("Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ="); httpClientGetRequest.println("Connection: close\r\n\r\n");
Please note that in the line above, I used the encoded string. This way, you are sending your username and password to the server.